What you need:
- A web server you control, with Apache, PHP and mod_php.
- The PEAR Mail package for PHP.
- An account on an SMTP-server. Many ISPs provide one of these for you.
The approach here is not to use any of the APIs (I counted to at least three, all supported by Blogger) that Blogger provide. Instead I took the easy way out and decided to use the e-mail "gateway" to create glob posts.
First, create the settings file:
<?php
// -----------------------------
// Configuration for newblog.php
// -----------------------------
// Password required to submit the post
$required_pass = "password";
// Blogger e-mail address. This is enabled and the secret
// configured in the admin interface at Blogger.com.
$to = "My blog <username.secret@blogger.com>";
// From address.
$from = "Me <my.address@somedomain.net>";
// The SMTP-server to use.
$host = "smtp.somedomain.net";
?>
Next, the actual code in newblog.php:
<html>
<body>
<p>
<?php
include("newblog_settings.php");
$method = $_SERVER['REQUEST_METHOD'];
if ($method == 'GET')
{
?>
<!-- Simple form. As light as possible. -->
<form name="newpost" action="newblog.php" method="post">
Password:
<br>
<input type="password" name="pass">
<br>
Subject:
<br>
<input type="text" name="subject">
<br>
Body:<br>
<textarea name="body"></textarea>
<br>
<input type="submit" value="Create post">
</form>
<script>
document.newpost.pass.focus();
</script>
<?php
} elseif ($method == 'POST')
{
$pass = $_POST['pass'];
if ($pass == $required_pass)
{
$subject = $_POST['subject'];
$body = $_POST['body'];
if ($subject == '' || $body == '')
{
echo "<p>Subject and body is required!</p>";
}
else
{
require_once "Mail.php";
$headers = array ('From' => $from,
'To' => $to,
'Subject' => $subject);
$smtp = Mail::factory('smtp',
array ('host' => $host,
'auth' => false));
// If authentication is needed, use this:
// $smtp = Mail::factory('smtp',
// array ('host' => $host,
// 'auth' => true,
// 'username' => 'user',
// 'password' => 'pass'));
$mail = $smtp->send($to, $headers, $body);
if (PEAR::isError($mail))
{
echo("<p>" . $mail->getMessage() . "</p>");
} else {
echo("<p>Message with subject \"" . $subject . "\" successfully sent!</p>");
}
}
}
else
{
echo("<p>Wrong password!</p>");
}
?>
<p><a href="/newblog.php">Create a new post</a></p>
<?php
}
?>
</p>
</body>
</html>
The code should be quite easy to understand so I won't get into any details here.
The password check is very basic but it should work well as long as no one is sniffing your network connection. And if they do I guess you could always enable SSL.
The result of running the script looks like this:

And after posting:

Resulting post:
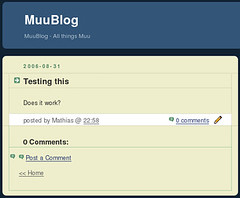
The screenshots are from a normal browser, but the result should look similar in even simple mobile phones that have a recent XHTML-browser.
There is one "bug" with the script and that is that characters outside ASCII (127 bit) will not be transferred correctly to the blog. I haven't figured out why, but I guess I would have to fiddle with MIME-encodings to get that working.
Happy blogging!
No comments:
Post a Comment